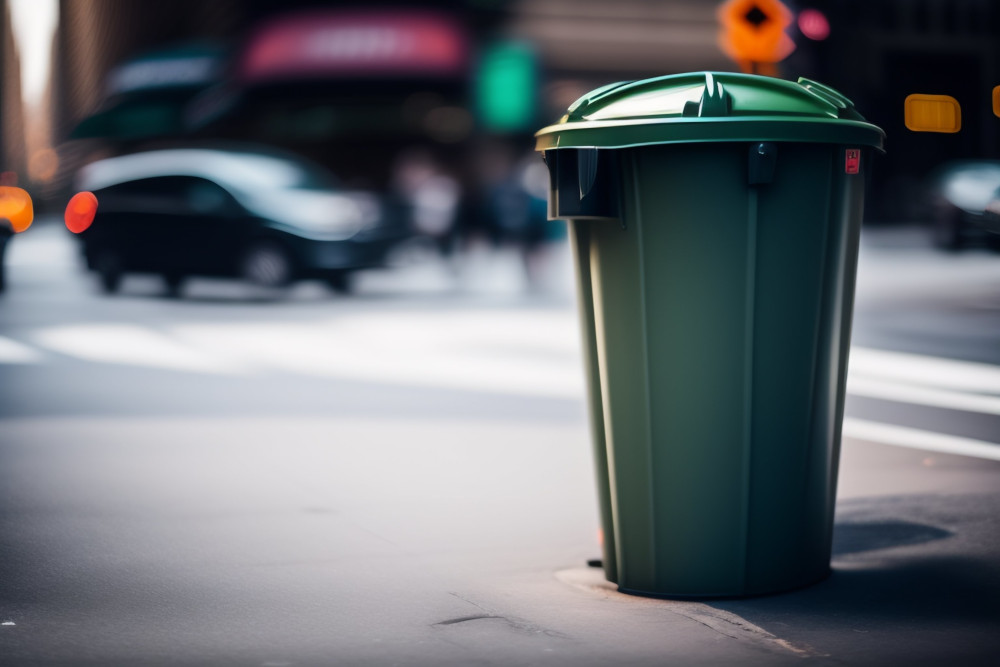
Delete Data fromGoogle Analytics 4
Google Analytics 4 provides two methods to delete data from a property – one through the GA4 admin interface and the other utilizing the Google Analytics User Deletion API. In this blog post, I will guide you through both methods, so you can choose the one that best suits your needs.
Method 1: Deleting Data from GA4 Interface
The GA4 admin interface provides a feature to create a “data deletion request.” In the admin menu, click on Data Deletion Requests to start the process.
The next screen shows an overview of data deletion requests that have been previously scheduled. Click on Schedule data deletion request to continue the process.
Next, you will be able to specify your request by choosing one of the five deletion types. For example, you can select Delete all parameters on all events to delete all data or define more specific conditions such as Delete selected registered parameters on selected events. This allows you to for example delete all values for the parameter transaction_id where event equals purchase. Unfortunately, it is currently not possible to delete data based on more complex conditions such as “delete all events where parameter hostname equals amazon.com”. That would be an awesome feature to delete spam or bug-related traffic retrospectively.
Finally, select the date range for your data deletion and click on Schedule request to complete the process.
Method 2: Deleting Data with the Google Analytics User Deletion API
If you need to delete data programmatically, the Google Analytics User Deletion API is the only available solution right now (February 2023). This API allows you to delete data for specific users from Google Analytics 4 properties. This section will show you how to use the API to delete data from a Google Analytics 4 property.
I will spoiler at this point: It is not feasible to fully erase all data from Google Analytics through this API. The deletion of a client id does impact certain metrics like the total user count, but it does not erase all events associated with that user. These events will persist in the system.
As I already mentioned, this API allows you to delete data only for specific users. If you are using a web property a user is identified with its client id. There are probably three ways how to get this id:
- User Explorer Report
- Custom Parameter
- Big Query
Option 1 works out of the box and does not require any extra setup. If you create a new report in the Explore section make sure that you select User Explorer as the template.
Relying on a report in the interface for a programmatic solution is not ideal but the client id is not (yet) available through the Google Analytics Data API. That’s where option 2 comes into play: Simply pass the Google Analytics Client Id from the _ga cookie to a new GA4 parameter. Now you can use it in a data API query.
Last but not least – Option 3: Use BigQuery to retrieve client ids by querying the user_pseudo_id column.
Once you have your client ids or know where to pull them from, get your Google Analytics 4 property id. I usually get it directly from the interface.
Once you have the credentials, you can use the following Python code to delete data from a Google Analytics 4 property. The code uses the Google Analytics API and the Google API Client Library for Python to delete a user from a Google Analytics 4 property.
from google.oauth2 import service_account
from googleapiclient.discovery import build
# Cache the authenticated service object for future use
_service = None
def get_analytics_service(scope):
"""
Returns an authenticated Google Analytics API service.
"""
global _service
# Check if the service has already been created and return it if it has
if _service:
return _service
# Load the credentials from the service account file
service_account_file = 'credentials/ga_api_credentials.json'
credentials = service_account.Credentials.from_service_account_file(service_account_file, scopes=scope)
# Create the service object and cache it
_service = build('analytics', 'v3', credentials=credentials)
return _service
def delete_ga4_user(id_type, user_id, property_id):
"""
Deletes a user from a Google Analytics 4 property.
"""
try:
# Get the authenticated Google Analytics API service
analytics_service = get_analytics_service(scope=["https://www.googleapis.com/auth/analytics.user.deletion"])
# Create the request body for the API request
body = {
"id": {
"type": id_type,
"userId": user_id
},
"propertyId": property_id,
"kind": "analytics#userDeletionRequest"
}
# Execute the API request to delete the user
response = analytics_service.userDeletion().userDeletionRequest().upsert(body=body).execute()
except Exception as e:
# Print an error message if there was an exception or error during the API request
print("Error deleting user: ", e)
return None
return response
# Call the delete_ga4_user function with your specific parameters
response = delete_ga4_user('CLIENT_ID', '11718400136.1675638735', '342183550')
# Print the response from the API request
print(response)